Python Fundamentals + CS Concepts
A One‑Stop Starter Class
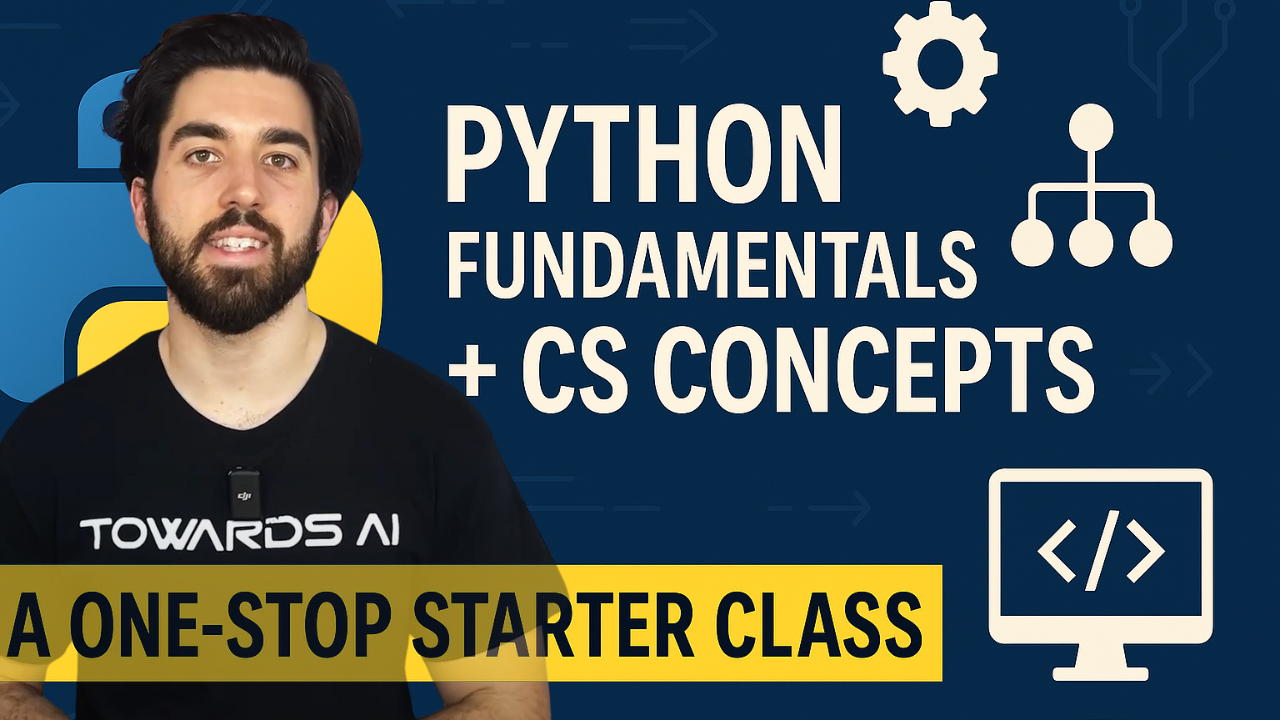
Watch the video:
See this article as a guide covering all the computer science fundamentals you need as a total programming beginner. We’ll go through all the concepts that are worth knowing about. Obviously, you’ll keep practicing and learn more about them in our Python course, so don’t worry if you don’t grasp all concepts from just this single article. You’ll need to practice with these programming concepts to really understand them!
Even if you leverage LLMs for coding, there are core computer science concepts that every programmer should know. We previously talked about programming languages and Python, but when coding, we also need a good sense of the fundamentals and how they fit into the bigger picture of software development. If you’re new to programming and feeling a bit unsure, don’t worry — I’ll take things one step at a time and use examples to explain each concept in a clear way
Let’s start by looking at the idea of syntax. Syntax means the formal rules that a programming language follows so the computer can understand your instructions. If you write code that breaks those rules, the computer will throw an error. Syntax is just like grammar in a human language. In English, you need a subject and a verb in most sentences. In Python, you need to write certain symbols or words in the right order for it to run. The important point is that learning syntax is about becoming comfortable with the way a programming language wants you to phrase your instructions. If you follow the rules, your code can actually do what you intend.
Programs also need a way to talk to the world and end users. An input is how you get new data into your program — like asking a user to type their name. An output is what your program shows back — like printing ‘Hello, World!’ to the screen. In Python, you’ll use input() to grab data and print() to display it, and these simple tools let your code interact with people or files.

But now, you might wonder how your coded instructions like this print function get turned into actions on your machine. This is the role of interpreters and compilers. An interpreter reads your code one chunk at a time and executes it on the fly. Python works this way, so when you run a Python script, the interpreter goes line by line, figuring out what you mean and then doing it. A compiler, by contrast, takes your entire code, analyzes it, and turns it into a separate program (usually machine code) that you can run later. C and C++ are common languages that use compilers. Ultimately, both interpreters and compilers turn your code into binary — 0s and 1s — that the computer’s hardware can understand, but you usually don’t need to worry about this low-level stuff! Instead, we want to understand what’s happening in front of our eyes, starting with those a and b things here we are printing to the screen and manipulating.

One of the most common rules you’ll learn about in a programming language is how to use a variable. A variable, here named a and b, are named containers that store data in your program with a clear label so that you can find it and use it again later. Well, in this case, it’s not so clear, but you may rather use something better like saying what it is actually storing. Think of it like a labeled box on a shelf. For instance, you could have a box labeled “favorite_number,” and inside it, you store the number 42. The concept of variables makes it straightforward to store, change, and reuse information whenever you need it. Variables also help your program keep track of its ‘state’ — the current snapshot of all the data it’s holding. If your favorite_number changes from 42 to 7, the state updates, and your program can act differently based on that new value.
Sometimes, though, you need to know what kind of data you’re putting in your variable box. This leads us to data types. A data type is a category that a piece of data fits into, such as integer, floating-point number, string, or boolean. Integers are whole numbers, floats are numbers with decimals, strings are sequences of characters like words or sentences, and booleans are values that are either true or false. Different languages handle data types differently, but the main idea is the same: It helps the computer know how to handle your data. For instance, adding two numbers is very different from trying to add a number and a sentence. Understanding data types is key to writing correct code.



Beyond these basic data types, Python also gives you ready-made containers to hold multiple pieces of data at once. These are called data structures — like lists (for ordered sequences, like [1, 2, 3]), dictionaries (for key-value pairs, like {“name”: “Alex”}), tuples (like lists but unchangeable), and sets (for unique items, like {1, 2, 3}). These help you to better organize your data in different ways, and you’ll use them all in your coding journey.
Once you have data types and structures under your belt, you’ll want to control what happens to your code under certain conditions. By default, Python runs your code from top to bottom, one line at a time. But sometimes you want to steer it in different directions or repeat steps — that’s where control structures like conditionals and loops come in. A conditional statement is a piece of code that runs only if a specific condition is met. It’s basically a way of saying, “If something is true, do one thing; otherwise, do something else.” A real-world example might be a security system that checks if you have the correct passcode to open a door. If the passcode is correct, the door unlocks. If it’s incorrect, an alarm goes off. Conditionals bring logic to your program and let you create branches in your workflow so your code can handle different situations.

Along similar lines, you may want your program to repeat a task multiple times. That’s where loops come into play. A loop is a construct that repeats a block of code until a certain condition is met or until it has done its work a set number of times. For example, if you want to print the numbers from 1 to 10, you can use a loop to do that automatically without typing each number’s print statement by hand. This not only saves you time but also reduces the chance of making mistakes. Loops are a major part of automating tasks and handling repetitive processes in your code.


Another concept you’ll likely bump into is functions. A function is a self-contained block of code that performs a specific task and can be reused by calling its name. The precise definition of a function is that it’s a named piece of code that takes inputs (which we often call parameters), processes them, and then returns an output. Functions help you organize your code better, avoid duplication, and keep things neat and readable. Instead of writing the same code in multiple places, you write it once inside a function and then call that function whenever you need it. They’re about performing actions and packaging them neatly.

Functions are just the start, however — Python also offers classes and objects to group data and actions into one unit. A class is a tool for defining something new in your program, mixing variables (like a user’s secret password) with functions that work on them (like saving or displaying that info). Objects are what you create from the class, each one carrying its own data but built from the same design. They’re about grouping data and actions into a single, reusable unit.

Now that we’ve seen how to break tasks into steps, let’s talk about the bigger picture: algorithms. An algorithm is a specific set of instructions for solving a problem or completing a task. If you write a recipe for baking cookies, you’re basically creating an algorithm. In coding, algorithms can be simple (like sorting a list) or very complex (like analyzing images). The key idea is that an algorithm outlines a step-by-step process for reaching a result. They’re about how to solve something, not the tools that do it. Once you grasp the idea of designing algorithms, you’re on your way to creating structured, efficient solutions for all kinds of problems.
While using functions, variables and objects and creating algorithms, there are two common principles that developers often talk about. One is DRY, which stands for “Don’t Repeat Yourself.” The main idea is to avoid writing the same piece of code multiple times in different places. If you need the same behavior, put it in a function or class, so basically in any of what we call our “shared resources”, and then call that function or resource wherever needed. This makes your code shorter, easier to read, and less prone to errors. The other principle is KISS, short for “Keep It Simple, Stupid.” This phrase is a reminder that sometimes, the simplest solution is the best one. The programmer’s version of Occam’s razor. It’s also to remember to avoid unnecessary complexity so that your code remains readable and maintainable. Another trick for readable code is adding comments — little notes you write with a # to explain what your code does. Starting with clear comments and tidy habits makes your code easier for you — and others — to work with later. Company leads will love you if you already document your code properly as a junior developer!
Without comments
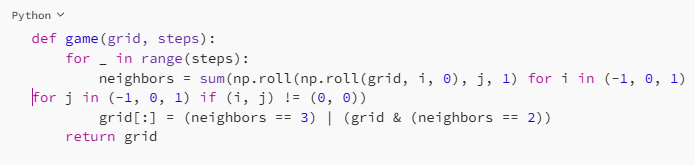
With comments
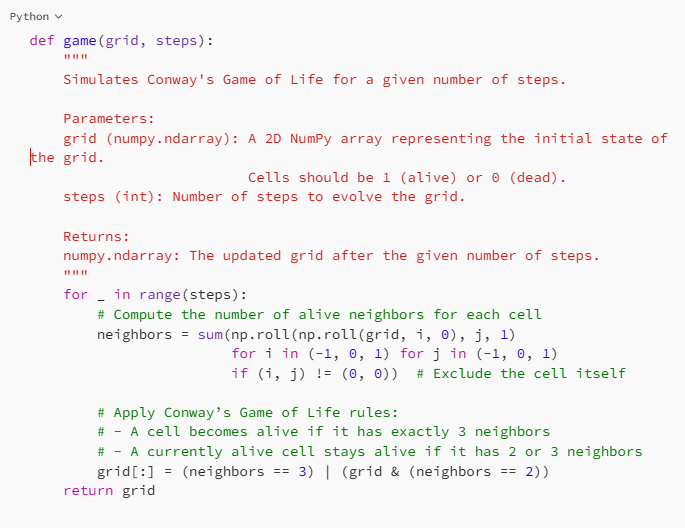
As you write more programs, you’ll find yourself wanting to use tools other people have created. We do that using libraries. A library is a collection of code other developers wrote to solve common problems. For example, there might be a library for working with dates, or a library for performing math operations. Instead of reinventing the wheel, you can just import a library and call the functions that someone else has already tested. Libraries are part of what makes programming such a friendly field: people share their solutions so you don’t have to start from scratch.
Without library
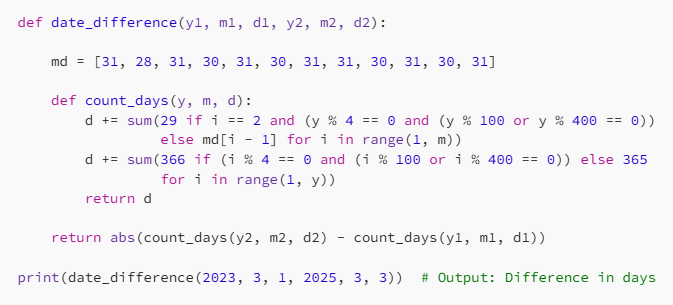
With library
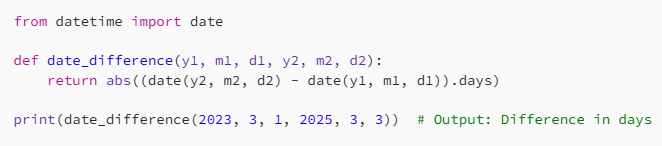
These extra libraries also become what we call dependencies. If you’re writing a program that uses a library to draw graphs, that library is a dependency because your code relies on it. When using libraries, especially in Python, you often install them with a package manager. In Python, the common one is pip. A package manager is a tool that automatically downloads and installs libraries, along with any libraries they depend on. Managing dependencies can get tricky since different projects might need different versions of the same library. That’s where pip shines — it grabs the right versions for you. Pip is like an app store for Python code, letting you grab the code you need from a central repository so you can use it in your project. The only problem is that if you have multiple projects going on, you may need different libraries that can have different conflicting dependencies, which can lead to a lot of issues. Trust me! To avoid that, you need to split these projects. This is done with virtual environments. A virtual environment does exactly that; it is a way to keep your project’s dependencies isolated from other projects on the same computer. It creates a small sandbox, so each project can have exactly the libraries and versions it requires without clashing with anything else.


Another step up from libraries is the concept of a framework. A framework is like a more comprehensive set of tools, libraries, and guidelines designed to help you build larger applications. For instance, in web development, frameworks like Django or Flask provide a lot of built-in code to handle user sessions, databases, and other common features. Using a framework can save you a lot of time because many essential parts of your application are already set up. You just fill in the details that are unique to your project.



Okay, so that was a lot! Feel free to listen to this again later on if it was too much. But, fortunately, now you know the core coding building blocks and how to access ready-made code from elsewhere, but you might by now be wondering: where do you actually go to write your code and how do you actually run it? That would be quite useful to know, right?
No matter what you’re building, you’ll need an environment to write your code. A code editor is a program that highlights your syntax and helps you write and manage files. It’s basically a text editor but with extra features for programmers, such as coloring keywords, auto-completing code, or integrating with tools like version control. Some people like the simplicity of a code editor because it’s lighter and faster for smaller tasks.
If you want extra features like visual debugging tools, project management, or advanced build processes, that’s when you use an IDE. An IDE, or Integrated Development Environment, is a software application that bundles a code editor with other utilities. It might analyze your code on the fly, suggest corrections, manage your dependencies, and let you step through your program line by line to see what’s happening. These features can be really helpful, especially as your projects become larger and you need a deeper understanding of what’s going on in your code. And now, a lot of these features are amazing AI-based improvements that we will also discuss in our course.
But writing code is only half the story — you also need a way to tell the computer to actually do what’s in the code, which is to run it. One option is to open a command-line terminal and type in commands that run your scripts directly. The command line (also known as the shell or terminal) might look a bit plain at first, but it’s incredibly powerful. You can create files, move them around, start programs, and pass in arguments all without leaving that single window. Many developers love the command line because it’s fast and doesn’t require a lot of overhead. Once you get accustomed to it, you can automate tasks and chain commands together in ways that feel almost magical. While many code editors and IDEs provide built in terminals and also support running code directly, others require external configuration or command-line execution.
CLI example
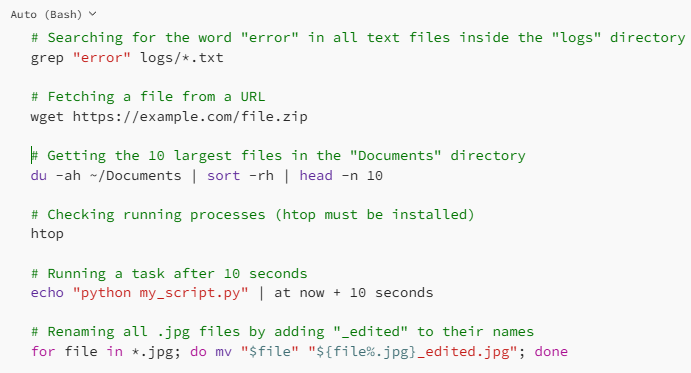
If you prefer a more interactive environment — especially for quick experimentation or data work with Python — Jupyter notebooks or Google Colab could be your cup of tea, as we’ll see. Notebook can run in your web browser, letting you write code in small chunks and see the results right away, often in text, plots, or even animations. It’s fantastic for exploring data, documenting steps, and sharing your process.
Depending on your goals, you might look into what we call containerization tools like Docker, which let you package your code and its environment together in one easily executable program, or explore continuous integration systems that automatically test and build your code every time you push a change. But for most beginners, a good grasp of the command line, a solid code editor or IDE, and an understanding of Jupyter notebooks or Google Colab is enough to cover most bases in day-to-day programming.
No matter which environment you choose, you’ll eventually make mistakes. That’s totally normal, and that’s where debugging comes in. Debugging is the process of finding and fixing those mistakes. It might involve reading error messages, using print statements, or stepping through your program with a debugger. It can be frustrating at times, but it’s also an essential skill for any developer. Debugging teaches you to understand your code’s flow and to anticipate where bugs might appear. For example Python has a tool called try-except that lets you say, ‘Try this code, but if something goes wrong — like a user typing a word instead of a number — catch the mistake and handle it calmly instead of crashing.’


Sometimes, though, a bug won’t immediately show up until you try all sorts of different ways of using your code. That’s why software testing is important. Software testing means writing extra code or using tools that check if your main code behaves as expected under different conditions. For instance, you might write tests, which we call unit tests, that see what happens when someone enters a valid password vs. an invalid one or when your function receives a number that’s much larger than usual. Testing reduces surprises and makes sure your program handles the variety of cases it might face in the real world.
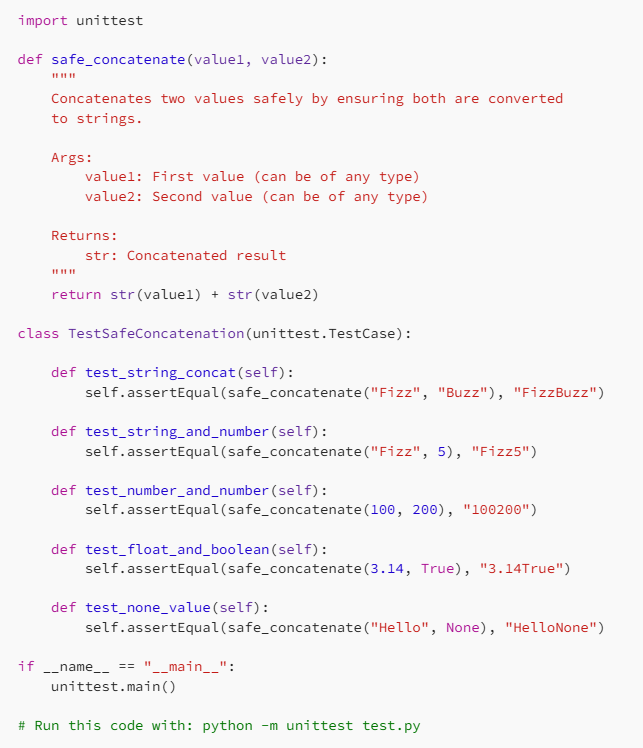
Even with testing, developers sometimes want the option to roll back to an older version of their code if something goes wrong. That’s where version control comes in. Version control is a system that tracks changes to your files over time so you can see what was changed and when. It also makes collaborating with others simpler because everyone can work on different parts of the code at once and then merge their changes together.

The most popular version control tool is Git. It’s a program you install on your computer that lets you commit your changes with a short message describing what you did. If you want to share your code with others, you can host it on a platform like GitHub. GitHub is a website that stores your Git repositories so that other people can view your code, collaborate with you, or even suggest changes. If you’re working in a team, Git and GitHub become central tools for managing your development process.
Sooner or later, especially if you’re curious about web development, you’ll hear about web servers. A web server is a program that listens for requests coming in over the internet and sends back responses. For example, when you type a website’s address into your browser, a web server receives that request, figures out what you’re looking for, and sends the web page back to your browser. This back-and-forth communication usually follows a protocol known as HTTP, which stands for Hypertext Transfer Protocol. An HTTP request might say, “Give me the homepage,” and the server replies, “Here it is.”

Sometimes, instead of getting a web page, you want data. Like when you are exchanging with a language model inside your code, for example. That’s where an API comes in. An API, or Application Programming Interface, is a set of rules and endpoints that let different programs talk to each other. If a website has an API, you can send it requests (like, “I need the current weather”) and get back data in a consistent format. APIs make it easier for different applications to connect and exchange information.

When working with data, you might want to store it somewhere so it doesn’t disappear when your program shuts down. That’s where a database comes in. A database is a system for organizing, storing, and retrieving data efficiently. There are many ways to store and manage data. The simplest is just to store it in a file, such as a CSV (comma-separated values) or JSON (JavaScript Object Notation) file. CSV files store data in a tabular form with each value separated by commas, while JSON structures data in key-value pairs that can be easily nested. More complex solutions include relational databases, like MySQL, which organize data into tables with rows and columns. There are also non-relational databases, such as MongoDB, which store data in documents or key-value pairs. You can interact with each type of database through your code, adding, editing, and querying data as needed.
Lastly, many modern projects run on servers that you don’t manage yourself. This is often called cloud computing. Cloud computing means using someone else’s hardware and services to deploy your applications, store files, or run databases. Companies like Amazon Web Services, Microsoft Azure, or Google Cloud let you rent servers by the month, hour or minute so you don’t have to maintain your own physical machines. It’s a handy approach for many teams because it’s easier to scale up or down depending on the traffic and resources you need.
These ideas — variables, loops, functions, and more — are the building blocks you’ll use to tell Python exactly what to do, whether you’re crunching numbers, building a game, or scraping a website. On top of that, tools and practices like libraries, frameworks, package managers, environments, debugging and testing are essential for actually writing and running code efficiently.
Alright, I know, this was a lot, but you are done with all the things to know. I hope this overview of core computer science ideas has helped you feel a bit more comfortable with the terminology you’ll see in your coding journey. These topics show up everywhere, from small scripts to huge applications. In the course, we’ll take these fundamentals and use them in projects that give you hands-on practice. Feel free to ask questions whenever you need more clarity. Thanks for sticking around, and I’ll see you next time.
