The Era of "ChatGPT Programming"
An Introductory Python Programming with ChatGPT
Watch the video:
Good morning, and welcome to this very first video lesson (linked above) of our Python course. It’s awesome to have you here, whether you’re someone who has dabbled in coding before or you’re just starting out with zero background in programming, computer science, or artificial intelligence. Maybe you heard about all these ChatGPT things and how they can help you write code, and you thought, “Hey, maybe I should learn the basics of coding while also exploring this AI angle.” If so, you’re in the right place. Today, I want to give you a friendly, down-to-earth introduction to what programming really is, what Python looks like, why coding might take some time to master, and how large language models (like ChatGPT) can help speed up your learning process.
First off, you might be wondering: what is programming, exactly? Some people imagine it as sitting at a computer, typing weird symbols and commands until you magically create the next Facebook. But really, programming is just a way of giving instructions to a computer so it can do things for you. Imagine the computer is an extremely literal person who only understands a very precise form of language. If you say, “Make me some coffee,” the computer will be clueless because that’s just plain English and general. Instead, you want to say something super specific, like “Boil 250 millilitres of water at 90 degrees Celsius, grind 15 grams of coffee beans, take a filter and put the powdered coffee in it, pour water over grounds for 30 seconds,” and so on.
Then, you can break down the first step into multiple atomic steps, such as: getting the pitcher, placing it under the faucet, turning the water on, waiting 10 seconds or until the water reaches 250 millilitres, turning the water off, etc.
With this simple example, we can see that (A) tasks can be divided into multiple smaller tasks and (B) you can aggregate multiple tasks into a single larger task and abstract away the tiny details.
These principles are two core building blocks of programming!
That’s basically what programming is. You’re breaking down tasks step by step, in a language the computer understands, so that you get the results you want.
Now, one reason programming might feel overwhelming at first is that computers can do a huge range of tasks. One moment you’re asking the computer to just display “Hello, World!” and the next you might want it to analyze a gigantic dataset or build an entire website. But don’t worry, you learn all of this in small steps. In general, you’ll see a pattern: identify the problem (like, “I want a greeting message”), plan a solution (like, “Okay, it needs to print a line of text to the screen”), write some code (maybe just a few lines), test it, and then refine it if there’s any bug or if you decide you want something fancier. This is the programming cycle — plan, code, test, refine — and you’ll see it repeat all the time from the first “Hello World” display to the final website.
Programming languages are the tools we use to communicate with the computer. You’ve probably heard of languages like Python, Java, C++, JavaScript. They all do the same fundamental thing — let you tell the computer what to do — just with slightly different flavors and syntaxes. Syntax is the set of rules you have to follow so the computer doesn’t get confused. If you mess up the syntax, the computer complains in the form of error messages. It’s kind of like if you tried to speak Spanish but mixed in random bits of French grammar. People might look at you like, “What are you even saying?” Computers are the same, except they complain a lot faster and more bluntly. No worries, though: the more you practice, the more naturally these rules will come to you. Just like regular languages.
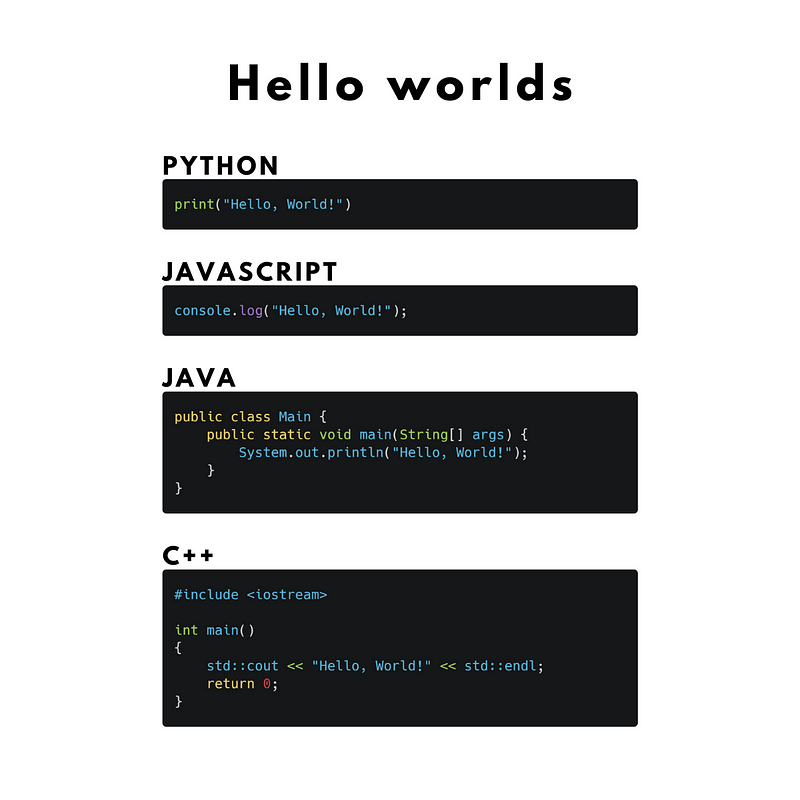
We’ll be focusing on Python in our course because it’s usually considered one of the friendliest languages for beginners, and most supported in the AI community. Python code often reads almost like English, which is part of its charm. For example, if you want to display the famous greeting “Hello, World!” on the screen, the Python code is literally just: print(“Hello, World!”). That’s one line. If you run it, you’ll see “Hello, World!” pop up. It’s simple, yet it shows you the entire process in miniature. You wrote an instruction, fed it to Python, Python understood it, and it displayed your message.
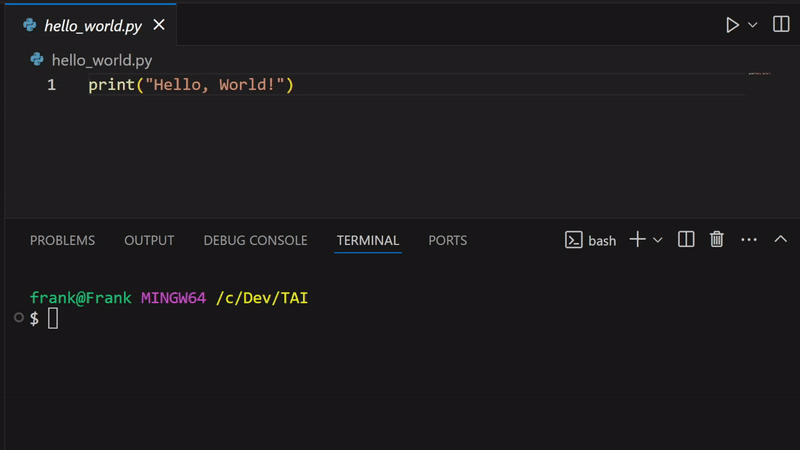
Now, you might be asking, if Python is so straightforward, why does it still take time to learn it? The truth is, while the basic stuff like print is super easy, Python also has variables, lists, dictionaries, functions, loops, conditionals, classes, modules, and a whole bunch of other features. You won’t become an expert overnight, just like you can’t learn all the ins and outs of Spanish or French or any new language in a single day. You need to dive deep into it and practice. On top of that, programming is about thinking like a problem-solver. You learn to break big problems down, consider different ways to approach them, test out solutions, handle errors, and keep improving. That’s a skill you develop over time. So don’t be discouraged if, at first, your code throws you a lot of error messages or if you don’t fully grasp how to structure a program. We’ve all been there, and it’s part of the journey.
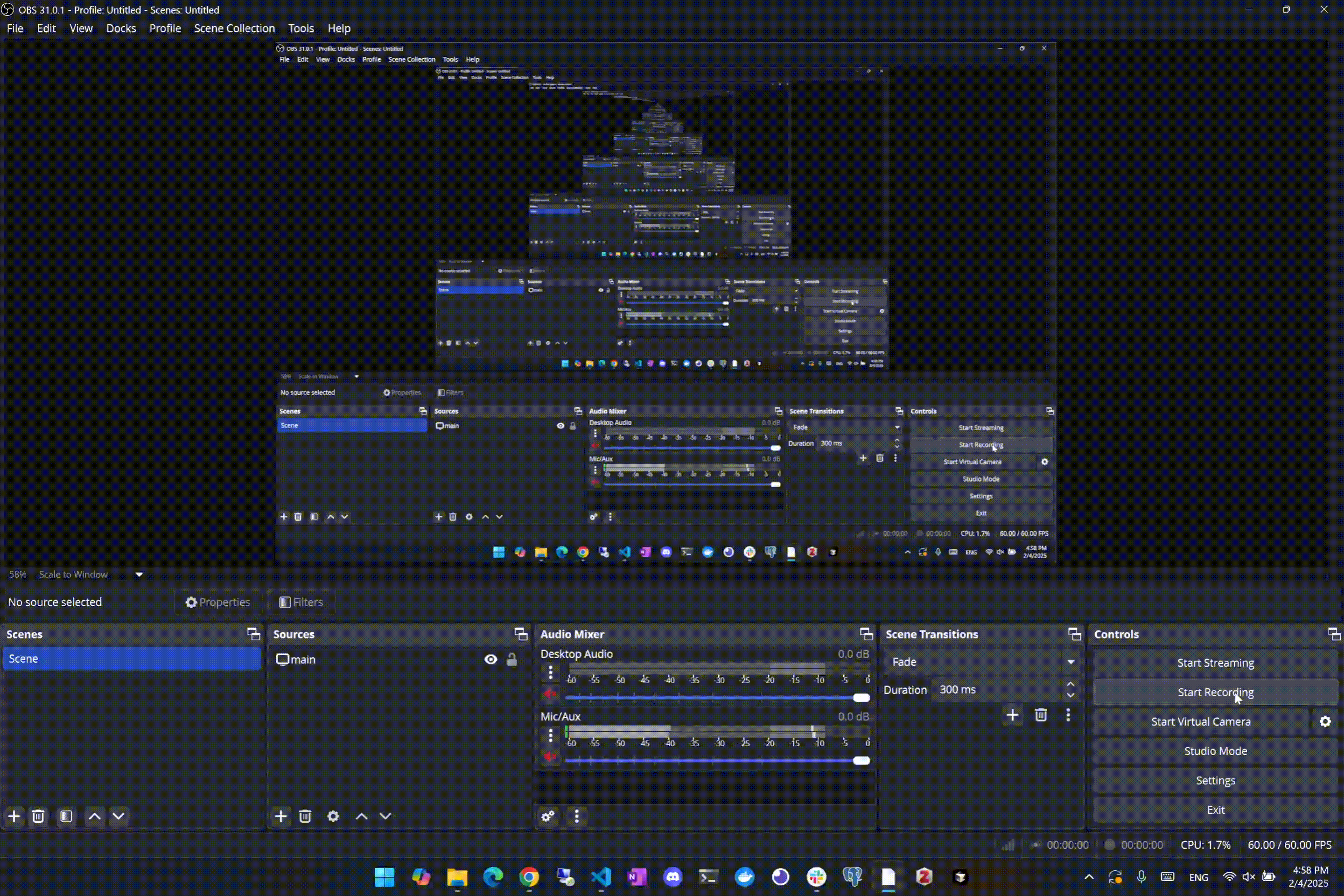
At this point, you might be wondering, so where do large language models like ChatGPT fit into all this? Well, ChatGPT and other LLMs are basically super-powerful text predictors. They were trained on tons of written data — think of them like someone who’s read nearly every article, book, website, and piece of code out there on the internet. When you type a question, they use all that training to guess what the best response might be, one word at a time. Because many of them have also been trained on code, they can write code, explain it, or help you fix it. It’s like having a super knowledgeable but not always perfect assistant by your side. You can say, “Hey ChatGPT, can you write a Python function that calculates the area of a circle?” and it’ll just spit out the code. If you’re confused about the code, you can say, “Okay, but can you explain what this line means?” and ChatGPT will walk you through it. Then maybe you decide you also want the program to handle negative inputs gracefully, so you ask ChatGPT to modify the code, and it will try to do that for you as well.
That’s one big reason they can be awesome teachers. They’re available anytime, they don’t complain if you ask the same question a thousand times, they don’t judge you and they can adapt to your pace. Sometimes, a traditional course will teach everything from the ground up: what a variable is, what a loop is, how to work with strings, and so on, before you get to make something fun. ChatGPT can turn that upside down. You can jump right into making a small game or a calculator, or a greeting program, get the code from ChatGPT, ask questions about it, and gradually learn the language as you go. We call this approach “top-down” learning. It’s a bit like learning to cook your favourite dish by diving right into the recipe rather than trying to memorize every single cooking technique first. If you need to know a technique while doing the recipe, you just google it or ask ChatGPT. It allows you to see results much faster and have fun, enjoying the process if you are a tinkerer like me. Both methods have merit, but ChatGPT unlocks that top-down style in a way that was much trickier before.
Let me give you a quick example. Suppose I want a program that asks a user for their name and then greets them. If I ask ChatGPT for a simple Python code that does that, it might give me a few lines like these ones…

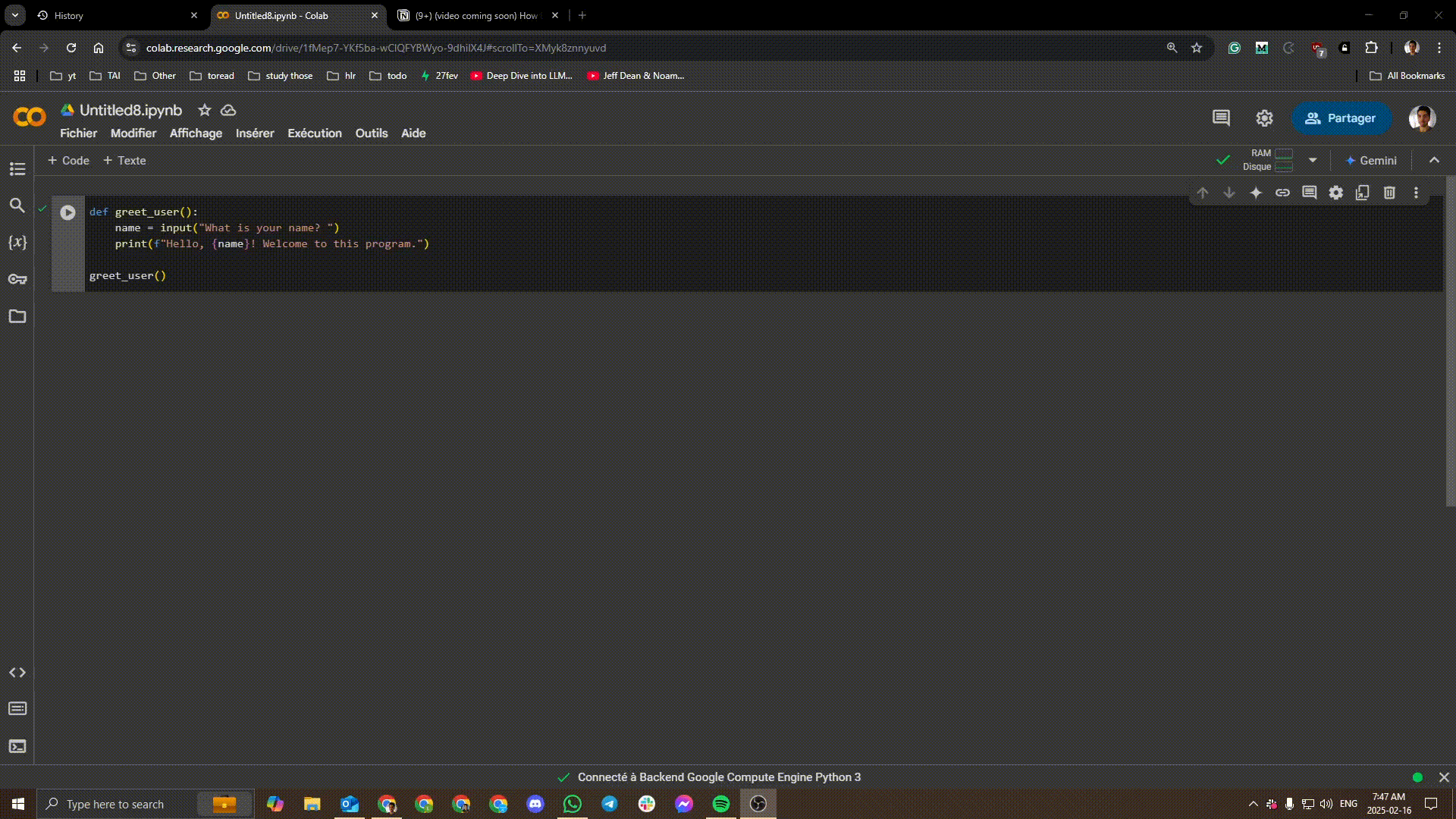
Then I’d copy-paste that into a file or an online Python environment, run it, see the prompt “What is your name?” and if I type “Alice,” it’ll respond, “Hello, Alice! Welcome to this program.” That’s a mini success. If I’m brand new, I might wonder how every line of the code works, so I’d go back to ChatGPT and say, “Explain how that code works line by line.” Then I’d read the explanation:
This code asks for your name, then says hello with your name included. First, it creates a “greet_user” task. Inside that task, it asks, “What is your name?” and saves your answer. Then, it uses your answer to say, “Hello, [your name]! Welcome.” Finally, it starts the task.
Next, I can say, “Now adjust it so it also tells the user how many letters are in their name.” ChatGPT might add a line that calculates the length of the name and prints that out. This is the top-down approach in action: I didn’t read tens of pages about Python’s syntax. I just asked for code, got an example, and learned from it.
One question you might have is: if ChatGPT can just write code for me, do I even need to learn how to program? The answer is undeniably yes, for a few reasons. First, ChatGPT isn’t perfect. It’s a brilliant tool, but it can make mistakes or misinterpret your instructions. You need to be able to catch errors, reason about your code, and figure out how to fix it when ChatGPT’s suggestions don’t work as planned. Second, you might want to customize your code in ways that aren’t straightforward for ChatGPT to guess. You might say, “Actually, I don’t want to just greet the user, I want to greet them in a rotating set of five languages, randomly chosen.” That’s the kind of problem-solving and creativity that’s more fun to figure out yourself, with ChatGPT helping along the way. Lastly, the real power of coding is what it does for your mind — it helps you develop logical thinking, a methodical approach to problem-solving, and an understanding of how the technologies shaping our world actually work. It’s one thing to talk about AI and coding; it’s another thing entirely to know what’s going on under the hood.
That’s why the best approach, in my opinion, is to see ChatGPT as your personal tutor rather than a magical code generator. Yes, it can generate code, but you should also ask it to explain how that code works. You can experiment with variations, read the error messages that come up, learn from them, and in general treat the AI as an interactive learning partner. That’s especially true when it comes to debugging. Debugging is the process of finding and fixing errors in your code. If your program crashes, it’s not a sign that you’re terrible at programming; it’s just part of the normal day-to-day life of any coder. Even the best programmers in the world spend a lot of time debugging. You’ll want to get comfortable with reading error messages, thinking logically about what might have gone wrong, and trying out fixes. ChatGPT can help you interpret those error messages and propose possible solutions. But you’ll learn the most when you start predicting the likely cause of the error yourself and verifying if ChatGPT’s fix actually makes sense.
Now, aside from just typing out instructions to ChatGPT in a browser, there’s another way to get code suggestions from AI: modern IDEs, or Integrated Development Environments. An IDE is basically a specialized interface that helps you write and run your code, offering features like syntax highlighting (where your code is colored in a way that’s easier to read), debugging tools, and project organization. Many IDEs now offer AI-assisted code completion. That means as you’re typing, the IDE is looking at your partial line of code and going, “Oh, I bet you want to type something like the print instruction next,” and it pops that suggestion in automatically. This can be a huge productivity boost, especially once you become a bit more comfortable with coding and can interpret the suggestions the AI is making. But again, you need at least a basic understanding of coding so you can tell if the suggestion is sensible. If you rely on it blindly, you might accept code that doesn’t do what you want.
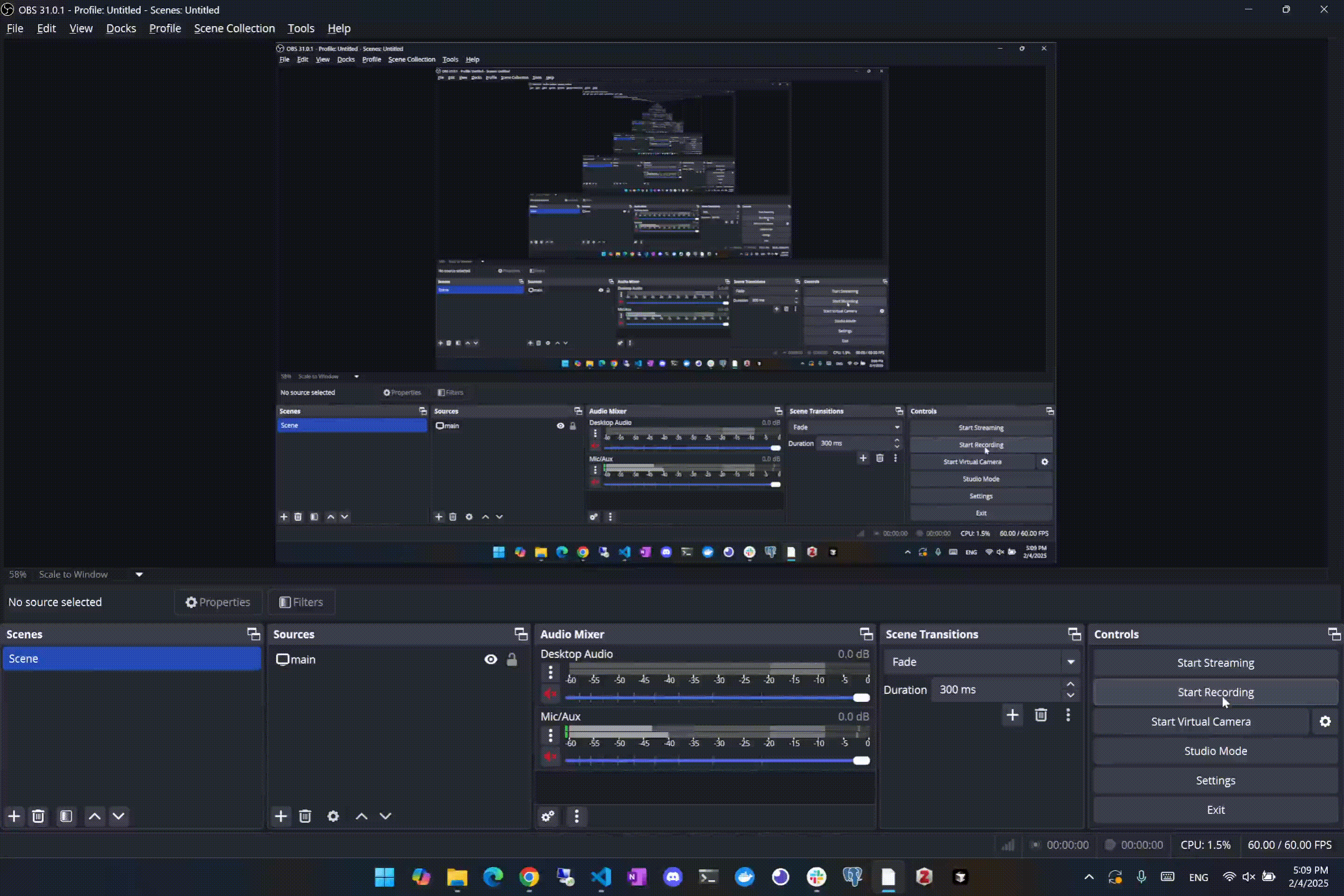
If you ever watch a more experienced coder work, you’ll notice that half of the job is thinking about what the code should do, planning the logic, figuring out how one piece interacts with another, and so on. Actually typing the code is only a small fraction of the job. That’s why tools like ChatGPT or other code completion engines can be so powerful. They speed up the mechanical side of coding, letting you focus on the creative and logical side. But it’s crucial to realize that you’re still the one steering the ship.
Of course, large language models aren’t perfect. Sometimes they generate code that looks plausible but has hidden flaws, or they might interpret your question incorrectly. They also don’t know anything that wasn’t in their training data, so if you ask for code that deals with very recent libraries or frameworks, you might find them missing some context. And from a security standpoint, you have to be careful if your code or data is sensitive. Don’t share your passwords or your clients’ personal data in a prompt to a public AI. Also, keep in mind the AI’s training data might include code that has licensing issues or questionable practices. So always double-check anything that’s mission-critical or that you plan to use in a professional context.
Still, these drawbacks shouldn’t scare you away from using LLMs as you learn. Just like any tool, you have to learn its strengths and weaknesses. It’s still pretty mind-blowing how fast you can generate prototypes, get quick explanations, or discover a new approach to a problem by interacting with the AI. The top-down approach becomes much more feasible and fun when you have an AI tutor right there to guide you. If you tried that approach back in the day, you’d have to rely on a big manual or you’d be stuck combing through hundreds of web pages, hoping to find an exact explanation that matched your scenario. Now you can just say, “Explain this error. Now fix the code. Now add this new functionality” and watch it adapt.
Another cool thing is that if you do want to eventually focus on the basics in a more systematic way (the bottom-up approach), you can still use the AI for that. You can ask it, “Teach me about variables,” or “Explain what a loop is in Python, with three examples.” It’ll happily walk you through it. I constantly ask it questions about new topics I learn about myself. So you have the freedom to jump between top-down exploration and bottom-up fundamentals whenever you want. This is great because everyone’s learning style is different. Some people prefer to see a finished product first, then dissect it. Others like to understand every detail from the ground up. You can do both, in whatever order suits you!
To wrap up, programming is this fascinating blend of creativity, logic, and problem-solving skills. Python is an excellent starting point because its syntax is simpler to read, and it’s super popular in fields like web development, scientific computing, data analysis, automation, and AI. Large language models like ChatGPT and the AI code completion in IDEs have transformed how we learn and practice programming. You are no longer stuck reading a giant textbook cover to cover to get started, even though it can be interesting, fun and definitely worthwhile sometimes. Still, you now have an alternative: you can build, explore, and tinker from day one, asking the AI for help whenever you hit a roadblock. Just keep in mind these new tools aren’t flawless and can mess up. Always practice debugging, verifying, refining and, most importantly, understanding your code. Over time, you’ll develop a solid mental model of how software works, making you more independent and more confident as you build your own projects.
That’s basically our game plan for this introduction to Python for AI course. We’ll encourage you to dive right in, have fun creating small programs and projects, and use ChatGPT or whatever LLM you prefer as your coding sidekick. The upcoming lessons will walk you through the fundamental concepts you need — how to store data in variables, how to loop over things, how to handle conditional logic, how to build bigger programs by organizing code into functions and modules, and so on. Meanwhile, you can keep asking the AI to explain what you don’t understand. If it seems confusing, you can even copy and paste your error messages and say, “Hey ChatGPT, what does this error mean, and how do I fix it?” The more you do this, the more these ideas will stick.
So, welcome again to the world of Python programming. This is just the beginning, and I’m excited for you to see how far you can go, especially with these AI tools at your disposal. You’ll be able to create interactive programs faster than you might have imagined and watch your coding skills grow. I can’t wait to see what you come up with in our final project. Let’s do this step by step, using that perfect combo of good old-fashioned learning, creative exploration, and some help from modern AI. Thanks for tuning in, and I’ll catch you in the next lesson.
If you are not enrolled yet, check out our Python course!
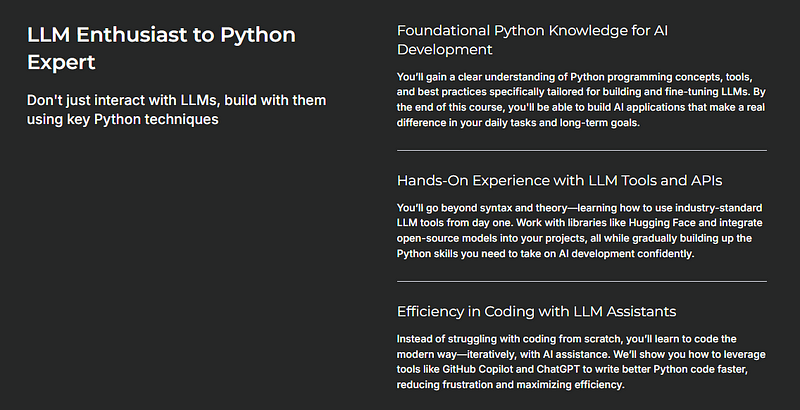